Git is easy
I have used git for quite a long time. I have also been coding in .NET for fairly some time now. I have created plenty of dotnet project repositories in Github and integrated some basic CI features to it using Github actions. It has all gone fairly well, until it didn’t, today.
The documentation is pretty solid and I know a lot of it on top of my head. But today, I ran into something that got me totally by surprise and wasted a lot of my time on it.
What was it?
I created a .NET Core project on Windows and gave it a mixed case name. I don’t know why I hadn’t thought of this biting me back later. Maybe it is because, when you are just working through exercises in a book, you do’t pay attention to such details.
I must have made like 30+ commits and pushed it to Github. I ignored the CI bit because, I thought it was just me, why do I care if it would successfully build on a remote server?
This was not a production application that I intended to deploy anywhere after all.
I created Marketplace while I followed some theory on Domain Driven Design reading and trying out concepts from Hands-On Domain Driven Design with .NET Core: Tackling Complexity in the heart of software by putting DDD principles into practice..
Did it need Continuous Integration?
Clearly this did not need CI. But would it hurt if it had?
If anything it would help me. I can only help me. It would tell me if I broke any tests before letting me merge my code in. So I took some time from my learning to setup a little CI Workflow using Github actions.
In case you were wondering what Github actions were, I have written a litle about it in another post where I talk about hosting a Hugo static generator blog on Azure storage.
So I chose to do it again, set up a github action to restore packages, build the project and run the tests. Simple right?
Simple workflow
I setup the yml file. Created a pull request. Triggered the action.
|
|
It could not be any simpler.
The action failed.
|
|
That was a very specific message about a file not being found.
Where was the file?
The file was right there!
I checked out the branch on my machine. I got all the files right. I had renamed the files earlier on my machine using traditional windows rename. And my machine always listed everything in the new case. I work on a Windows machine, so files can be named fully lowercase or mixed case or however you wish to. The OS doesn’t really care; it considers all names the same irrespective of the casing combination you choose.
I then checked Github.
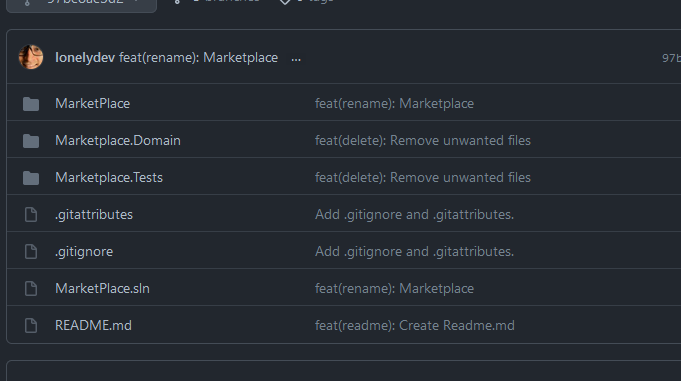
You must have spotted the problem already. But my eyes hadn’t.
I spend several minutes, I think almost more than an hour before I spotted the filename hadn’t changed on Github. In this case the directory name MarketPlace
was the culprit.
Could I have avoided this problem?
No amount of renaming on the operating system would help you in this case.
I tried renaming the folder in Git bash on Windows. Git does not even understand that a file rename happened.
I tried opening up Windows Subsystem for Linux and doing the rename in there.
Git, ignored all my attempts at rename.
I realised, that Git did not follow any of my operating system level rename operations. This gave me a hint! Something was telling get to ignore case sensitivity.
After some reading online I figured there was a configuration that did this.
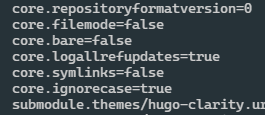
core.ignorecase
was the problem.
On a Windows 10 PC I had left it to true. So no matter how I rename files, when the source and destination names differed only in casing, git would ignore my change, as if it never happened!
Apparently, the right thing to do was to set this to true
on Windows since I began working on git hosted projects. This I clearly had not done.
|
|
This is great if you have not done any renames in the past already and pushed the changes to your remote. However, if you did rename in the past, DO NOT CHANGE THIS SETTING IN BETWEEN.
How do I fix this now?
That was my predicament.
There is a solution, of course.
A two step solution. Rename your MixedCase
file to something like anothername
, fully lowercased. Then rename it back to the correct casing. And to do this, you use git mv
and not just a regular rename (mv
).
|
|
That triggered Git to track my rename!
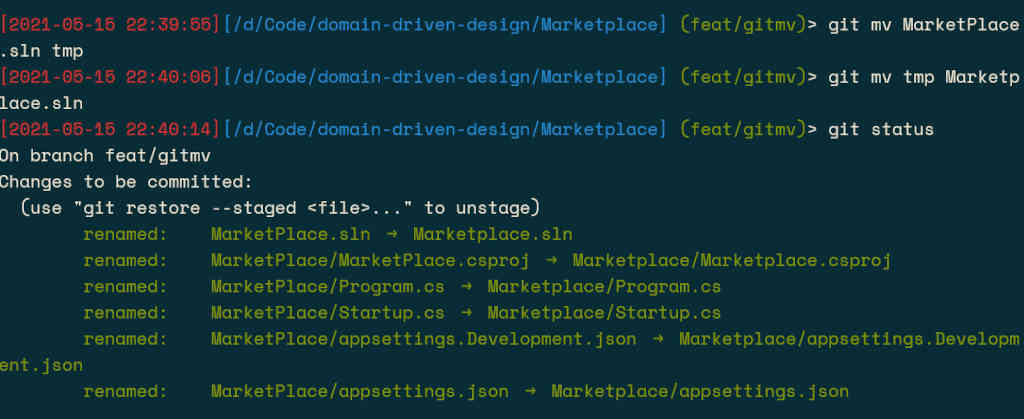
Once that was done, I could then rename the actual csproj
file too in the same way.
After the changes were ready, I committed my changes and voila! The github actions just worked.
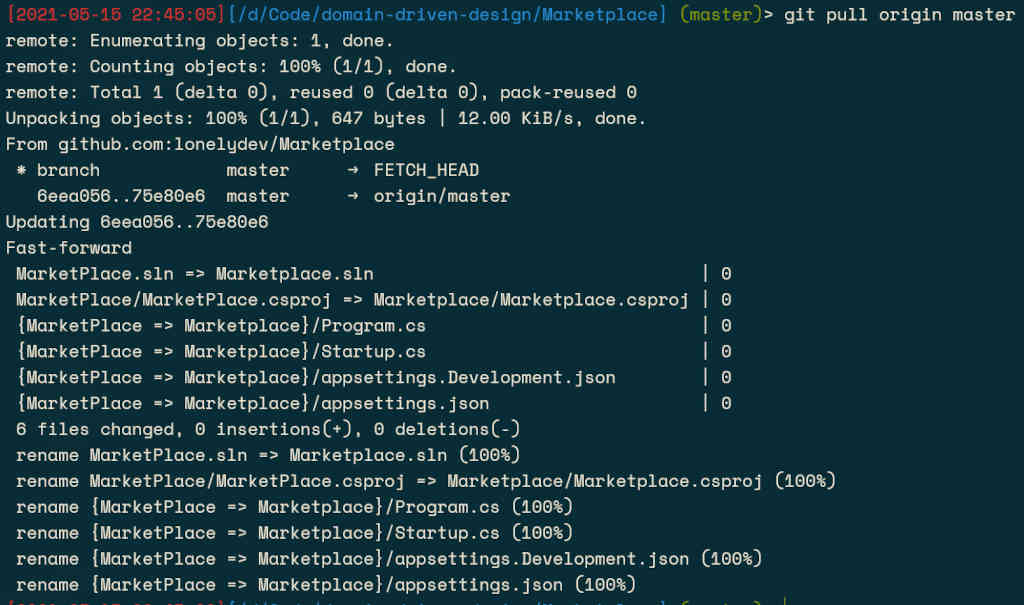
I hope that saved you some time.